Introduction
With its versatility and readability, Python is extensively favored for varied programming duties. One frequent operation inside this versatile language entails changing an inventory of strings into particular person components. This functionality facilitates environment friendly knowledge manipulation, offers flexibility in dealing with numerous datasets, and enhances general programming productiveness. This complete information will discover varied strategies and strategies to transform listing to string Python. We may also talk about the advantages, challenges, and greatest practices related to this conversion.
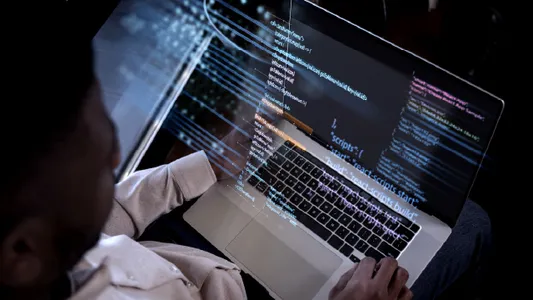
Understanding the Want: Convert Checklist to String Python
String lists include a number of components separated by commas and enclosed inside sq. brackets. Whereas strings are helpful for representing knowledge, they lack the flexibleness and performance of lists. To transform listing to string Python, we will carry out operations similar to indexing, slicing, appending, and modifying the weather extra simply.
Advantages to Convert Checklist to String Python
There are a number of advantages to changing a string listing to an inventory in Python.
- Firstly, it permits us to entry particular person components throughout the listing, enabling us to carry out particular operations.
- Secondly, lists are mutable, that means we will modify their components, whereas strings are immutable.
- Lastly, lists present a variety of built-in features and strategies that may be utilized to govern the information effectively.
Strategies for Changing: Convert Checklist to String Python
A number of strategies can be found to transform listing to string Python. Let’s discover a few of the mostly used strategies:
Utilizing the eval() Perform
The eval() operate in Python evaluates the expression handed to it as a parameter. It will possibly convert a string listing to an inventory by merely passing the string listing because the parameter to the eval() operate. Right here’s an instance:
Code
string_list = "[1, 2, 3, 4, 5]"
list_result = eval(string_list)
print(list_result)
Output
[1, 2, 3, 4, 5]
Utilizing the ast.literal_eval() Perform
The ast.literal_eval() operate is a safer different to the eval() operate. It evaluates the expression handed to it as a parameter however solely permits the analysis of literals similar to strings, numbers, tuples, lists, dicts, booleans, and None. Right here’s an instance:
Code
import ast
string_list = "[1, 2, 3, 4, 5]"
list_result = ast.literal_eval(string_list)
print(list_result)
Output
[1, 2, 3, 4, 5]
Utilizing Checklist Comprehension
Checklist comprehension is a concise solution to create lists in Python. It can be used to transform a string listing to an inventory by iterating over every aspect within the string listing and appending it to a brand new listing. Right here’s an instance:
Code
string_list = "[1, 2, 3, 4, 5]"
list_result = [int(x) for x in string_list[1:-1].cut up(",")]
print(list_result)
Output
[1, 2, 3, 4, 5]
Utilizing the cut up() Technique
The cut up() technique in Python splits a string into an inventory of substrings based mostly on a specified delimiter. We are able to convert a string listing to an inventory utilizing the cut up() technique and specifying the delimiter as a comma. Right here’s an instance:
Code
string_list = "[1, 2, 3, 4, 5]"
list_result = string_list[1:-1].cut up(", ")
print(list_result)
Output
[‘1’, ‘2’, ‘3’, ‘4’, ‘5’]
Utilizing Common Expressions
Common expressions present a strong solution to match and manipulate strings. We are able to extract the person components from the string listing and convert them into an inventory utilizing common expressions. Right here’s an instance:
Code
import re
string_list = "[1, 2, 3, 4, 5]"
list_result = re.findall(r'd+', string_list)
list_result = [int(x) for x in list_result]
print(list_result)
Output
[1, 2, 3, 4, 5]
Right here is the tabular comparability that can help you with convert listing to string Python
Method | Efficiency | Syntax and Readability | Error Dealing with |
Checklist Comprehension | Reasonable | Concise and Readable | Restricted error dealing with. Might elevate exceptions for invalid syntax. |
cut up() Technique | Reasonable | Concise and Readable | Restricted error dealing with. Assumes well-formatted enter. |
eval() Perform | Typically Sooner | Might result in safety dangers on account of executing arbitrary code | Higher error dealing with. Can deal with invalid syntax successfully, however potential safety issues. |
ast.literal_eval() Perform | Typically Sooner | Safer than eval(), evaluates literals solely | Higher error dealing with. Appropriate for literals, might not deal with all circumstances. |
Common Expressions | Reasonable to Gradual (Relying on Sample Complexity) | Could also be much less readable for advanced patterns | Restricted error dealing with. Relies on the correctness of the regex sample. |
Widespread Challenges and Errors: Convert Checklist to String Python
We might encounter sure challenges and errors whereas changing a string listing to an inventory. Let’s talk about a few of them:
Dealing with Invalid Syntax Errors
If the string listing accommodates invalid syntax, similar to lacking commas or brackets, it could lead to a SyntaxError. We are able to use error-handling strategies similar to try-except blocks to catch and deal with the errors gracefully.
Coping with Nested Lists
The conversion course of turns into extra advanced if the string listing accommodates nested lists. We should make sure that the nested lists are correctly evaluated and transformed to keep up the specified construction.
Addressing Reminiscence and Efficiency Points
Changing giant string lists to lists can devour important reminiscence and affect efficiency. It is very important optimize the conversion course of through the use of environment friendly strategies and strategies.
Finest Practices to Convert Checklist to String Python
To make sure a easy and environment friendly conversion course of, it is strongly recommended to observe these greatest practices:
Validating Enter Information
It is very important validate the enter knowledge earlier than changing a string listing to an inventory. This consists of checking for legitimate syntax, making certain the presence of mandatory delimiters, and dealing with any potential edge circumstances.
Error Dealing with and Exception Dealing with
Implement sturdy and exception dealing with mechanisms to deal with errors or exceptions in the course of the conversion course of. It will assist present significant error messages and stop program crashes.
Optimizing Efficiency
Optimize the efficiency of the conversion course of by selecting probably the most environment friendly technique based mostly on the particular necessities. Contemplate components similar to reminiscence utilization, execution time, and scalability whereas deciding on the suitable technique.
Writing Readable and Maintainable Code
Write code that’s simple to learn, perceive, and keep. Use significant variable names, add feedback the place mandatory, and observe coding conventions to boost the readability of the code.
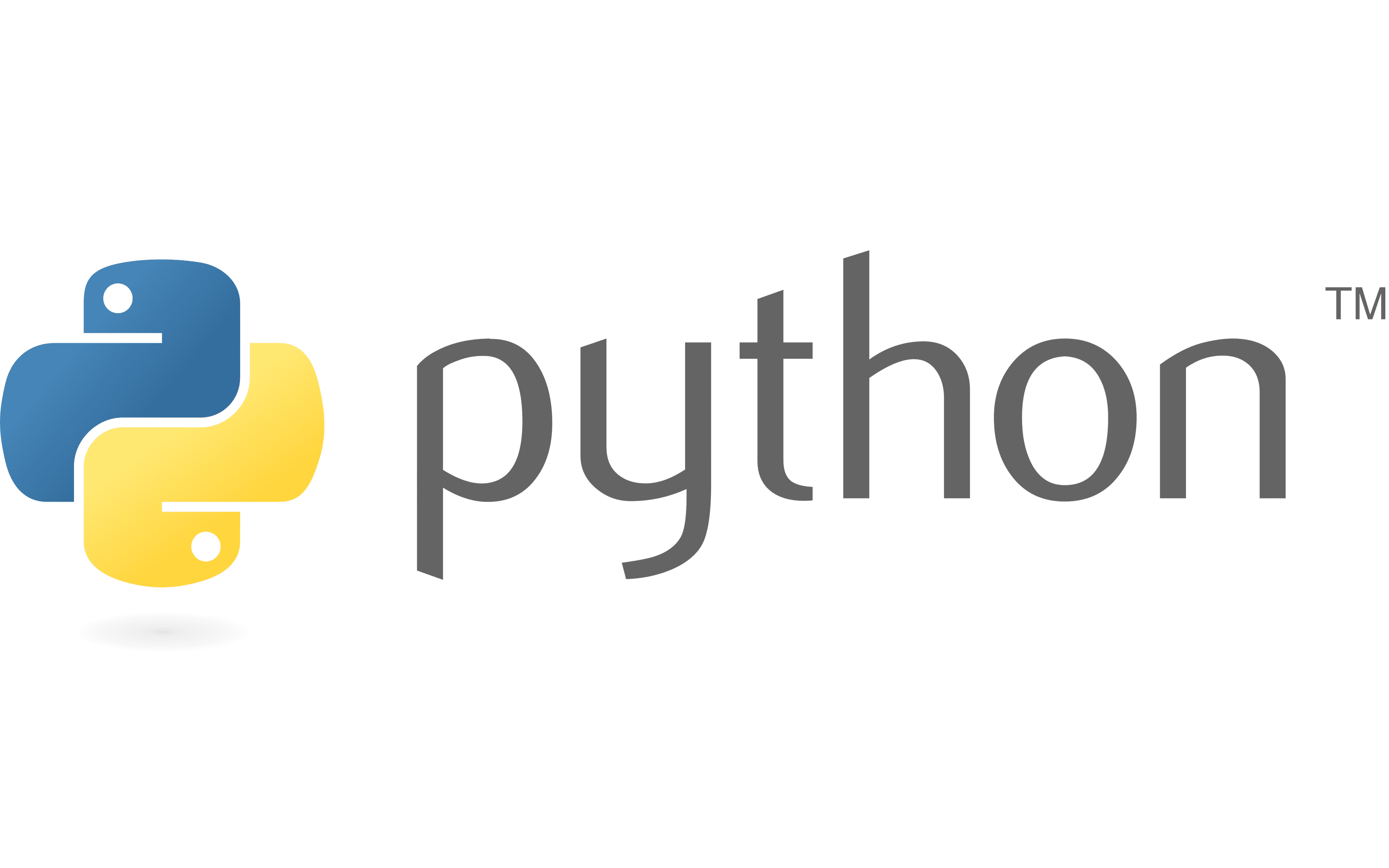
Comparability of Totally different Approaches to Convert Checklist to String Python
Let’s examine the totally different approaches mentioned above based mostly on efficiency, syntax, and error dealing with:
Efficiency Comparability
- Checklist Comprehension: Reasonable efficiency, appropriate for small to medium-sized datasets.
- cut up() Technique: Reasonable efficiency, environment friendly for easy circumstances, however might degrade with advanced strings.
- eval() Perform: Typically quicker however poses safety dangers. Cautious utilization is suggested.
- ast.literal_eval() Perform: Typically quicker, safer than eval(), appropriate for literals.
- Common Expressions: Reasonable to sluggish; efficiency will depend on the complexity of the sample.
Syntax and Readability Comparability
- Checklist Comprehension: Concise and readable, Pythonic strategy.
- cut up() Technique: Concise and readable, appropriate for easy circumstances.
- eval() Perform: Code might be concise however raises safety issues.
- ast.literal_eval() Perform: Concise and safe, appropriate for literals.
- Common Expressions: Could also be much less readable, particularly for advanced patterns.
Error Dealing with Comparability
- Checklist Comprehension: Restricted error dealing with might elevate exceptions for invalid syntax.
- cut up() Technique: Restricted error dealing with, assumes well-formatted enter.
- eval() Perform: Higher error dealing with, can deal with invalid syntax successfully, however poses safety dangers.
- ast.literal_eval() Perform: Higher error dealing with, appropriate for literals, might not deal with all circumstances.
- Common Expressions: Restricted error dealing with will depend on the correctness of the regex sample.
Select the strategy that aligns along with your necessities, contemplating efficiency, readability, and error-handling capabilities.
Conclusion
Changing a string listing to an inventory is a elementary operation in Python programming. By understanding the varied strategies, challenges, and greatest practices related to this conversion, we will successfully manipulate and work with knowledge extra effectively. Whether or not it’s changing a easy string listing to an inventory or a fancy string listing of lists to an inventory of lists, Python offers us with varied strategies to perform the duty. We are able to select probably the most appropriate technique by following one of the best practices and contemplating the particular necessities.
Need to sharpen your understanding of Python language? Be part of the Licensed AI & ML BlackBelt Plus Program and acquire complete insights into Python’s capabilities for knowledge science. Elevate your expertise and unlock new prospects in AI and machine studying. Enroll now to grow to be a proficient Python developer!