Introduction
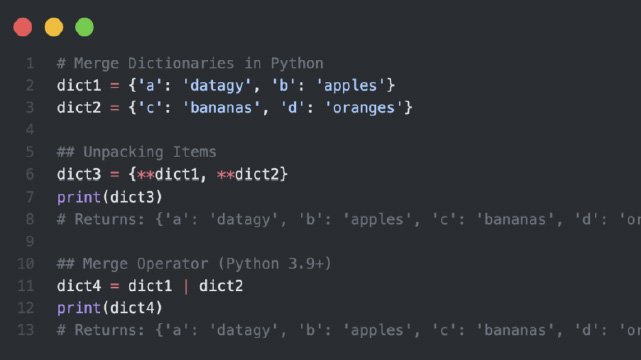
Dictionary merging is a typical operation in Python that enables us to mix the contents of two dictionaries right into a single dictionary. This course of is useful after we need to consolidate information from a number of sources or when we have to replace the values of current keys. This text will discover numerous strategies for merging dictionaries, talk about frequent situations the place merging is required, and supply greatest practices for dealing with key conflicts and preserving information integrity.
Strategies for Merging Dictionaries
There are a number of strategies out there in Python for merging dictionaries. Let’s discover every of those strategies intimately:
Utilizing the replace() Methodology
The replace() methodology is a built-in methodology in Python dictionaries that enables us to merge the contents of 1 dictionary into one other. This methodology takes one other dictionary as an argument and updates the calling dictionary with the key-value pairs from the argument dictionary. If there are overlapping keys, the values from the argument dictionary will overwrite the values within the calling dictionary.
Code:
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
dict1.replace(dict2)
print(dict1)
Output:
{‘a’: 1, ‘b’: 3, ‘c’: 4}
Utilizing the ** Operator
In Python, the `**` operator can merge two dictionaries. This operator unpacks the key-value pairs from the second dictionary and provides them to the primary dictionary. If there are any overlapping keys, the values from the second dictionary will overwrite the values within the first dictionary.
Code:
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
merged_dict = {**dict1, **dict2}
print(merged_dict)
Output:
{‘a’: 1, ‘b’: 3, ‘c’: 4}
Utilizing the dict() Perform
The dict() perform can even merge dictionaries in Python. This perform takes an iterable of key-value pairs as an argument and returns a brand new dictionary containing these pairs. By passing the key-value pairs from each dictionaries as arguments to the `dict()` perform, we are able to merge the dictionaries.
Code:
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
merged_dict = dict(dict1, **dict2)
print(merged_dict)
Output:
{‘a’: 1, ‘b’: 3, ‘c’: 4}
Utilizing the collections.ChainMap Class
The collections.ChainMap class in Python gives a method to merge dictionaries whereas preserving the unique dictionaries. This class creates a single view of a number of dictionaries, permitting us to entry and modify the merged dictionary as if it had been a single dictionary.
Code:
from collections import ChainMap
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
merged_dict = ChainMap(dict1, dict2)
print(merged_dict)
Output:
ChainMap({‘a’: 1, ‘b’: 2}, {‘b’: 3, ‘c’: 4})
Widespread Situations for Merging Dictionaries
Merging dictionaries is a flexible operation that may be utilized to numerous situations. Let’s discover some frequent situations the place merging dictionaries is helpful:
Merging Dictionaries with Overlapping Keys
When merging dictionaries, overlapping keys are anticipated. In such instances, the values from the second dictionary will overwrite the values from the primary dictionary. This enables us to replace the values of current keys or add new ones and values to the merged dictionary.
Code:
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
merged_dict = {**dict1, **dict2}
print(merged_dict)
Output:
{‘a’: 1, ‘b’: 3, ‘c’: 4}
Merging Dictionaries with Totally different Knowledge Sorts
Python dictionaries can include values of various information sorts, corresponding to strings, numbers, lists, and even different dictionaries. When merging dictionaries with totally different information sorts, the merged dictionary will include the values from each dictionaries, preserving their authentic information sorts.
Code:
dict1 = {'a': 1, 'b': [2, 3]}
dict2 = {'b': (4, 5), 'c': 'good day'}
merged_dict = {**dict1, **dict2}
print(merged_dict)
Output:
{‘a’: 1, ‘b’: (4, 5), ‘c’: ‘good day’}
Greatest Practices for Merging Dictionaries
When merging dictionaries in Python, following some greatest practices to make sure information integrity and keep away from potential points is important. Let’s talk about these greatest practices:
Dealing with Key Conflicts
When merging dictionaries, it’s anticipated to come across key conflicts the place the identical key exists. To deal with crucial conflicts, we should determine whether or not to overwrite the worth from the primary dictionary with the worth from the second dictionary or hold each values. This resolution depends upon the precise necessities of our software.
Preserving Order in Merged Dictionaries
By default, dictionaries in Python don’t protect the order of key-value pairs. Nonetheless, if order preservation is important, we are able to use the collections.OrderedDict class or third-party libraries like ordereddict or sortedcontainers to create ordered dictionaries. This ensures that the order of key-value pairs is maintained throughout the merging course of.
Avoiding Knowledge Loss throughout Merging
When merging dictionaries, you will need to guarantee no information is misplaced. To keep away from information loss, we should always rigorously take into account the merging methodology and deal with key conflicts appropriately. Moreover, it’s a good follow to create a backup of the unique dictionaries earlier than merging them in case we have to revert the modifications.
Comparability of Dictionary Merging Methods
Let’s evaluate the totally different methods for merging dictionaries based mostly on their efficiency, syntax, and use instances:
Efficiency Comparability
The efficiency of dictionary merging methods can fluctuate relying on the dimensions of the dictionaries and the variety of crucial conflicts. The replace() methodology and the `**` operator are typically sooner than the dict() perform and the collections.ChainMap class. Nonetheless, the efficiency distinction is probably not vital for small dictionaries.
Syntax Comparability
The syntax for merging dictionaries utilizing the replace() methodology and the `**` operator is concise and simple. Alternatively, the syntax for merging dictionaries utilizing the dict() perform and the collections.ChainMap class is barely extra verbose. The selection of syntax depends upon private choice and the applying’s particular necessities.
Use Case Comparability
The selection of dictionary merging approach depends upon the precise use case. If we have to replace the values of current keys or add new keys and values to an current dictionary, the replace() methodology or the `**` operator are appropriate decisions. Suppose we need to protect the unique dictionaries and create a single view of a number of dictionaries, the collections.ChainMap class is a greater possibility.
Conclusion
Merging dictionaries in Python is a strong operation that enables us to mix information from a number of sources and replace the values of current keys. This text explored numerous strategies for merging dictionaries, mentioned frequent situations the place merging is required, and supplied greatest practices for dealing with key conflicts and preserving information integrity. By understanding the totally different methods and their trade-offs, we are able to simply merge dictionaries in Python and manipulate information.