Introduction
Python features are an important a part of any programming language, permitting us to encapsulate reusable blocks of code. One essential facet of features is the flexibility to cross arguments, that are values that we offer to the perform for it to work with. On this definitive information, we are going to discover the varied forms of perform arguments in Python and learn to use them successfully.
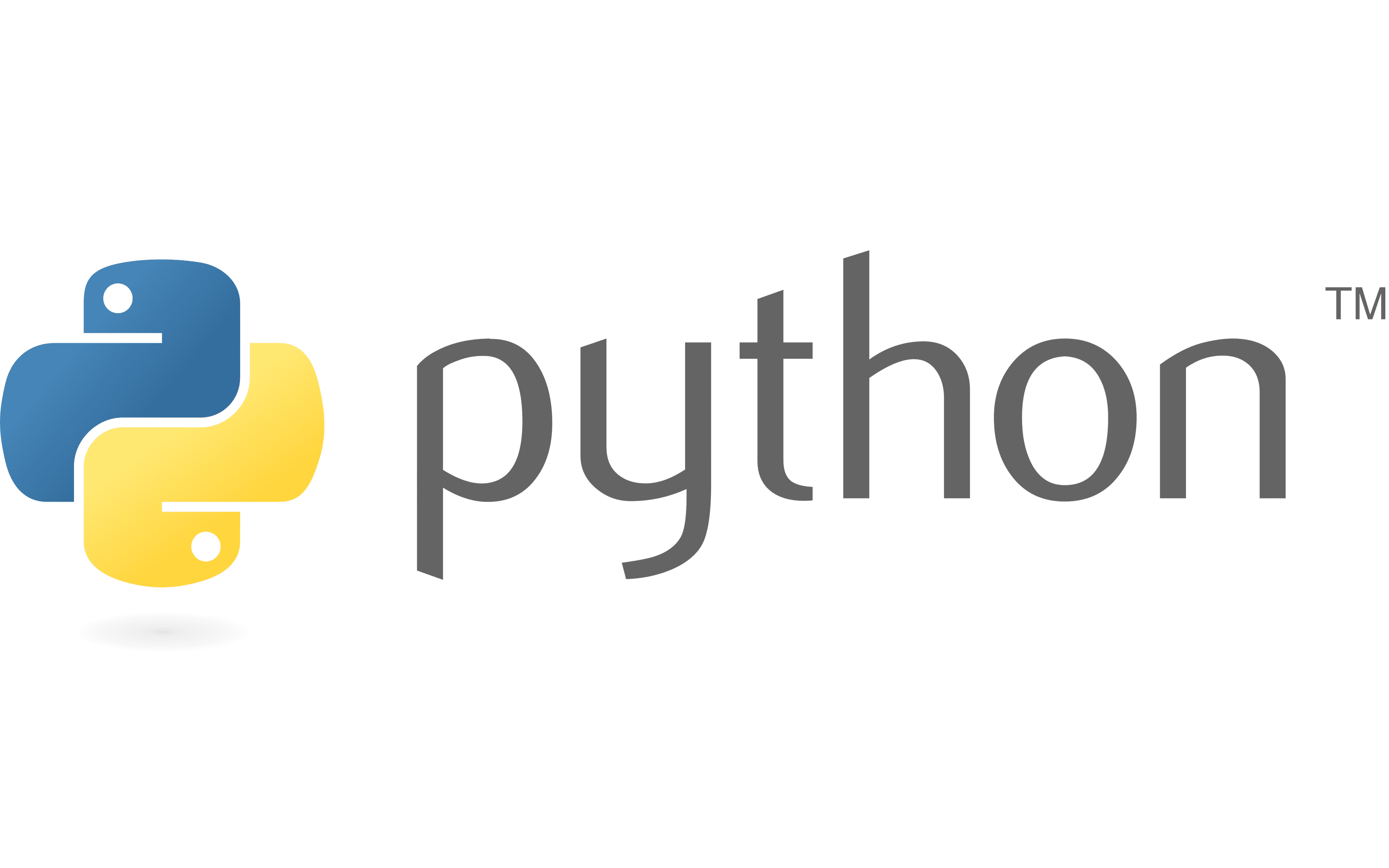
Understanding Python Perform Arguments
Python perform arguments play a vital position in creating versatile and environment friendly code. With positional, key phrase, and default arguments, builders can tailor features to various wants. Perform arguments are the values that we cross to a perform once we name it. These arguments present the required inputs for the perform to carry out its job. Python helps several types of perform arguments, together with positional arguments, key phrase arguments, and arbitrary arguments.
Positional Arguments
Positional arguments are essentially the most primary sort of Python perform arguments. They’re outlined within the perform signature and are handed within the order they’re outlined. Let’s discover totally different features of positional arguments.
Single Positional Argument
A perform can have a number of positional arguments. A single positional argument is outlined by specifying its identify within the perform signature. For instance
Code:
def greet(identify):
print(f"Good day, {identify}!")
greet("Alice")
On this instance, the `greet` perform takes a single positional argument, `identify`. Once we name the perform with the argument `”Alice”`, it prints “Good day, Alice!”.
A number of Positional Arguments
A perform may have a number of positional arguments. These arguments are separated by commas within the perform signature. For instance:
Code:
def add_numbers(a, b):
return a + b
end result = add_numbers(3, 5)
On this instance, the `add_numbers` perform takes two positional arguments, `a` and `b`. Once we name the perform with the arguments `3` and `5`, it returns the sum of the 2 numbers: ‘ 8`.
Default Values for Positional Arguments
Python permits us to assign default values to positional arguments. These default values are used when the caller doesn’t present a worth for the argument. For instance:
Code:
def greet(identify="World"):
print(f"Good day, {identify}!")
greet() # Output: Good day, World!
greet("Alice") # Output: Good day, Alice!
On this instance, the `greet` perform has a positional argument `identify` with a default worth of `”World”`. Once we name the perform with out arguments, it makes use of the default worth and prints “Good day, World!”. If we offer an argument, akin to `”Alice,”` it makes use of the offered worth and prints “Good day, Alice!”.
Unpacking Positional Arguments
Python offers a method to unpack an inventory or tuple and cross its parts as positional arguments to a perform. That is helpful when now we have a set of values we wish to cross as separate arguments. For instance:
Code:
def add_numbers(a, b):
return a + b
numbers = [3, 5]
end result = add_numbers(*numbers)
On this instance, now we have an inventory of `numbers` containing the values `[3, 5]`. Utilizing the `*` operator earlier than the listing, we unpack its parts and cross them as positional arguments to the `add_numbers` perform. The result’s `8`, the sum of `3` and `5`.
Key phrase Arguments
Key phrase arguments are one other sort of perform argument in Python. Not like positional arguments, key phrase arguments are recognized by their names relatively than their positions. Let’s discover totally different features of key phrase arguments.
Single Key phrase Argument
A perform can have a number of key phrase arguments. A single key phrase argument is outlined by specifying its identify and default worth within the perform signature. For instance:
Code:
def greet(identify="World"):
print(f"Good day, {identify}!")
greet() # Output: Good day, World!
greet(identify="Alice") # Output: Good day, Alice!
On this instance, the `greet` perform has a key phrase argument `identify` with a default worth of `”World”`. We will name the perform with none arguments, and it makes use of the default worth. Alternatively, we will present a worth for the `identify` argument explicitly utilizing its identify.
A number of Key phrase Arguments
A perform can have a number of key phrase arguments. These arguments are outlined by specifying their names and default values within the perform signature. For instance:
Code:
def calculate_area(size=1, width=1):
return size * width
space = calculate_area(size=5, width=3)
On this instance, the `calculate_area` perform has two key phrase arguments, `size` and `width,` with default values of `1`. We will name the perform and explicitly present values for these arguments utilizing their names. The result’s `15`, the product of `5` and `3`.
Default Values for Key phrase Arguments
Just like positional arguments, we will assign default values to key phrase arguments. These default values are used when the caller doesn’t present a worth for the argument. For instance:
Code:
def greet(identify="World"):
print(f"Good day, {identify}!")
greet() # Output: Good day, World!
greet(identify="Alice") # Output: Good day, Alice!
On this instance, the `greet` perform has a key phrase argument `identify` with a default worth of `”World”`. Once we name the perform with out arguments, it makes use of the default worth and prints “Good day, World!”. If we offer an argument, akin to `”Alice”,` it makes use of the offered worth and prints “Good day, Alice!”.
Unpacking Key phrase Arguments
Python offers a method to unpack a dictionary and cross its key-value pairs as key phrase arguments to a perform. That is helpful when now we have a set of values we wish to cross as separate key phrase arguments. For instance:
Code:
def greet(identify):
print(f"Good day, {identify}!")
individual = {"identify": "Alice"}
greet(**individual)
On this instance, now we have a dictionary `individual` containing the key-value pair `{“identify”: “Alice”}.` Utilizing the `**` operator earlier than the dictionary, we unpack its key-value pairs and cross them as key phrase arguments to the `greet` perform. The output is “Good day, Alice!”.
Arbitrary Arguments
Python permits us to outline features that may settle for a variable variety of arguments. These are often called arbitrary arguments and are useful when we have to know what number of arguments might be handed to the perform. There are two forms of arbitrary arguments: `*args` for variable-length positional arguments and `**kwargs` for variable-length key phrase arguments.
*args: Variable-Size Positional Arguments
The `*args` syntax permits us to cross a variable variety of positional arguments to a perform. The arguments are collected right into a tuple, which might be accessed throughout the perform. Let’s see an instance:
Code:
def add_numbers(*args):
whole = 0
for num in args:
whole += num
return whole
end result = add_numbers(1, 2, 3, 4, 5)
On this instance, the `add_numbers` perform accepts any variety of positional arguments utilizing the `*args` syntax. The arguments `1, 2, 3, 4, 5` are collected right into a tuple `args`. We then iterate over the tuple and calculate the sum of all of the numbers: ‘ 15`.
**kwargs: Variable-Size Key phrase Arguments
The `**kwargs` syntax permits us to cross a variable variety of key phrase arguments to a perform. The arguments are collected right into a dictionary, which might be accessed throughout the perform. Let’s see an instance:
Code:
def greet(**kwargs):
for key, worth in kwargs.objects():
print(f"{key}: {worth}")
greet(identify="Alice", age=25, metropolis="New York")
On this instance, the `greet` perform accepts any variety of key phrase arguments utilizing the `**kwargs` syntax. The arguments `”identify”: “Alice”,` `”age”: 25`, and `”metropolis”: “New York”` are collected right into a dictionary `kwargs.` We then iterate over the dictionary and print every key-value pair.
Mixing Completely different Varieties of Arguments
Python permits us to combine several types of arguments in a perform. We will have positional, key phrase, and arbitrary arguments in the identical perform signature. Let’s discover some examples.
Positional Arguments with Key phrase Arguments
A perform can have each positional arguments and key phrase arguments. The positional arguments are outlined first, adopted by the key phrase arguments. For instance:
Code:
def greet(identify, age=30):
print(f"Good day, {identify}! You're {age} years previous.")
greet("Alice") # Output: Good day, Alice! You're 30 years previous.
greet("Bob", age=25) # Output: Good day, Bob! You're 25 years previous.
On this instance, the `greet` perform has a positional argument `identify` and a key phrase argument `age` with a default worth of `30`. We will name the perform with simply the `identify` argument, and it makes use of the default worth for `age.` Alternatively, we will explicitly present a worth for `age` utilizing its identify.
Key phrase Arguments with Positional Arguments
A perform may have key phrase arguments adopted by positional arguments. The key phrase arguments are outlined first, adopted by the positional arguments. For instance:
Code:
def greet(age=30, identify):
print(f"Good day, {identify}! You're {age} years previous.")
greet(identify="Alice") # Output: Good day, Alice! You're 30 years previous.
greet(age=25, identify="Bob") # Output: Good day, Bob! You're 25 years previous.
On this instance, the `greet` perform has a key phrase argument `age` with a default worth of `30` and a positional argument `identify.` We will name the perform with simply the `identify` argument, and it makes use of the default worth for `age.` Alternatively, we will explicitly present a worth for `age` utilizing its identify.
Mixing Positional Arguments, Key phrase Arguments, and Arbitrary Arguments
Python permits us to combine positional, key phrase, and arbitrary arguments in the identical perform. The positional and key phrase arguments are outlined first, adopted by arbitrary arguments. For instance
Code:
def greet(identify, age=30, *args, **kwargs):
print(f"Good day, {identify}! You're {age} years previous.")
print("Extra arguments:")
for arg in args:
print(arg)
print("Extra key phrase arguments:")
for key, worth in kwargs.objects():
print(f"{key}: {worth}")
greet(" Alice," 25, " Good day," " World," metropolis=" New York," nation=" USA ")
On this instance, the `greet` perform has a positional argument `identify,` a key phrase argument `age` with a default worth of `30`, and arbitrary arguments `*args` and `**kwargs.` We will name the perform with the `identify` and `age` arguments, adopted by extra positional and key phrase arguments. The output consists of the identify, age, extra positional, and key phrase arguments.
Greatest Practices for Utilizing Perform Arguments
When utilizing perform arguments in Python, following some finest practices to make sure clear and maintainable code is important. Let’s discover a few of these finest practices.
Selecting Descriptive Argument Names
When defining perform arguments, selecting descriptive names that convey their function is essential. This makes the code readable and helps different builders perceive the perform’s habits. For instance:
Code:
def calculate_area(size, width):
return size * width
On this instance, the arguments `size` and `width` clearly point out the realm’s dimensions being calculated.
Maintaining the Variety of Arguments Affordable
Maintaining the variety of arguments in a perform cheap is mostly really useful. Extra arguments should be made to make the perform simpler to grasp and use. If a perform requires many inputs, it could be an indication that it’s doing an excessive amount of and ought to be refactored into smaller, extra centered features.
Documenting Python Perform Arguments
Documenting the Python perform arguments is important to make it simpler for different builders (together with your future self) to grasp and use your features. This may be accomplished utilizing docstrings and multi-line feedback instantly after the perform signature. For instance:
Code:
def greet(identify):
"""
Greets the desired identify.
Args:
identify (str): The identify to greet.
"""
print(f"Good day, {identify}!")
On this instance, the docstring clearly describes the `identify` argument and its anticipated sort.
Avoiding Mutable Default Argument Values
When defining default values for Python perform arguments, avoiding utilizing mutable objects akin to lists or dictionaries is mostly really useful. It is because mutable default values are shared throughout a number of perform calls, resulting in sudden habits. As an alternative, use immutable objects or `None` as default values and deal with mutable objects throughout the perform. For instance:
Code:
def add_item(merchandise, objects=None):
if objects is None:
objects = []
objects.append(merchandise)
return objects
On this instance, the `add_item` perform takes an `merchandise` argument and an non-compulsory `objects` argument, which defaults to `None.` If `objects` is `None,` we create a brand new empty listing. This ensures that every perform name has its listing for storing objects.
Utilizing Kind Hints for Python Perform Arguments
Python helps sort hints, which permit us to specify the anticipated forms of perform arguments. Kind hints enhance code readability and may also help catch potential type-related errors. For instance:
Code:
def calculate_area(size: float, width: float) -> float:
return size * width
On this instance, the kind hints `float` point out that the `size` and `width` arguments ought to be floating-point numbers. The return sort trace `float` signifies the perform will return a floating-point quantity.
Widespread Errors and Pitfalls with Python Perform Arguments
When working with Python perform arguments, it’s necessary to grasp the widespread errors and pitfalls that may happen with perform arguments. This part will discover a few of these errors and supply examples that will help you keep away from them.
Forgetting to Present Required Arguments
One widespread mistake is forgetting to offer the required arguments when calling a perform. This will result in errors and sudden habits. Let’s think about an instance:
Code:
def greet(identify):
print(f"Good day, {identify}!")
greet() # Error: lacking 1 required positional argument: 'identify'
Within the above instance, the `greet()` perform requires a `identify` argument. Nonetheless, once we name the perform with out offering any arguments, Python raises an error indicating {that a} required argument is lacking.
All the time present the required arguments when calling a perform to keep away from this error.
Misusing Default Argument Values
Python permits us to outline default values for perform arguments. Nonetheless, misusing default argument values can result in sudden outcomes. Contemplate the next instance:
Code:
def multiply(a, b=2):
return a * b
end result = multiply(3)
print(end result)
# Output: 6
Within the above instance, the `multiply()` perform has a default argument `b` set to 2. Once we name the perform with just one argument (`multiply(3)`), Python makes use of the default worth for `b` and returns the results of multiplying 3 by 2.
To keep away from misusing default argument values, rigorously think about the habits of your perform and select acceptable default values.
Overusing Arbitrary Arguments
Python permits us to outline features with arbitrary arguments utilizing the `*args` syntax. Whereas this may be helpful in sure conditions, greater than utilizing arbitrary arguments could make the code simpler to grasp and keep. Let’s see an instance:
Code:
def calculate_average(*numbers):
whole = sum(numbers)
common = whole / len(numbers)
return common
end result = calculate_average(1, 2, 3, 4, 5)
print(end result) # Output: 3.0
Within the above instance, the `calculate_average()` perform accepts any variety of arguments and calculates their common. Whereas this may be handy, different builders might not know what the perform expects as enter.
To keep away from overusing arbitrary arguments, think about using express arguments when doable and solely use `*args` when obligatory.
Ignoring Argument Order
One other widespread mistake is ignoring the order of arguments when calling a perform. This will result in sudden outcomes and bugs in your code. Let’s think about an instance:
Code:
def divide(a, b):
return a / b
end result = divide(2, 4)
print(end result) # Output: 0.5
end result = divide(4, 2)
print(end result) # Output: 2.0
Within the above instance, the `divide()` perform expects two arguments: `a` and `b.` If we ignore the order of the arguments when calling the perform, we are going to get totally different outcomes.
To keep away from this error, all the time present the arguments within the right order when calling a perform.
Not Dealing with Sudden Arguments
Python permits us to outline features with numerous arguments utilizing the `**kwargs` syntax. Nonetheless, not dealing with sudden arguments can result in errors and sudden habits. Let’s see an instance:
Code:
def print_person_info(identify, age):
print(f"Title: {identify}")
print(f"Age: {age}")
print_person_info(identify="John", age=25, metropolis="New York")
Within the above instance, the `print_person_info()` perform expects two arguments: `identify` and `age.` Nonetheless, we additionally present a further argument, `metropolis.` For the reason that perform doesn’t deal with sudden arguments, Python raises an error.
To keep away from this error, both deal with sudden arguments explicitly or use key phrase arguments to offer extra info.
Superior Python Perform Argument Ideas
Along with the widespread errors and pitfalls, Python offers superior ideas for working with perform arguments. On this part, we are going to discover a few of these ideas.
Argument Unpacking with *
Python permits us to unpack an inventory or tuple and cross its parts as particular person arguments to a perform utilizing the `*` operator. This may be helpful when working with variable-length argument lists. Let’s think about an instance:
Code:
def multiply(a, b, c):
return a * b * c
numbers = [2, 3, 4]
end result = multiply(*numbers)
print(end result) # Output: 24
The above instance defines an inventory `numbers` containing three parts. By utilizing the `*` operator earlier than the listing when calling the `multiply()` perform, Python unpacks the listing and passes its parts as particular person arguments.
Argument Unpacking with **
Like argument unpacking with `*,` Python additionally permits us to unpack a dictionary and cross its key-value pairs as key phrase arguments to a perform utilizing the `**` operator. Let’s see an instance:
Code:
def print_person_info(identify, age):
print(f"Title: {identify}")
print(f"Age: {age}")
individual = {"identify": "John", "age": 25}
print_person_info(**individual)
The above instance defines a dictionary `individual` containing two key-value pairs. By utilizing the `**` operator earlier than the dictionary when calling the `print_person_info()` perform, Python unpacks the dictionary and passes its key-value pairs as key phrase arguments.
Argument Annotation and Kind-checking
Python permits us to annotate perform arguments with sorts utilizing the `:` syntax. Whereas the interpreter doesn’t implement these annotations, they are often helpful for documentation and type-checking instruments. Let’s think about an instance:
Code:
def greet(identify: str) -> None:
print(f"Good day, {identify}!")
greet("John")
Within the above instance, we annotate the `identify` argument of the `greet()` perform with the kind `str`. This means that the perform expects a string argument. Whereas Python doesn’t implement this kind, type-checking instruments can use these annotations to catch potential sort errors.
Perform Argument Decorators
Python permits us to outline decorators, which modify the habits of different features. We will use decorators so as to add extra performance to perform arguments. Let’s see an instance:
Code:
def uppercase_arguments(func):
def wrapper(*args, **kwargs):
args = [arg.upper() for arg in args]
kwargs = {key: worth.higher() for key, worth in kwargs.objects()}
return func(*args, **kwargs)
return wrapper
@uppercase_arguments
def greet(identify):
print(f"Good day, {identify}!")
greet("John")
The above instance defines a decorator `uppercase_arguments` that converts all arguments to uppercase earlier than calling the embellished perform. By making use of the `uppercase_arguments` decorator to the `greet()` perform, we modify its habits to greet the individual in uppercase.
Conclusion
On this definitive information to Python perform arguments, now we have coated numerous features of working with perform arguments. We explored widespread errors, pitfalls, and superior ideas akin to argument unpacking annotation and interior designers.
You’ll be able to write extra strong and maintainable Python code by understanding these ideas and avoiding widespread errors. All the time present the required arguments, use default values correctly, and deal with sudden arguments appropriately.
Able to supercharge your profession? Be a part of our unique information science course and energy up with Python, the trade’s main language. No coding or information science background? No drawback! This course is tailored for freshmen such as you. Seize the chance to launch your information science journey immediately. Don’t miss out on this opportunity to amass beneficial Python abilities that can set you aside. Enroll now and take step one towards a profitable and rewarding profession in information science!
Proceed your Python journey by exploring extra about perform arguments and experimenting with totally different examples. Blissful coding!